Integration with HTTPS and JSONata
Description
In the context of an e-commerce purchase, consider an integration that manages the payment processing through the following steps:
-
Order reception
-
Purchase information retrieval
-
Data transformation
-
Payment processing
-
Status update
-
Final response
See the complete script and the integration’s diagram.
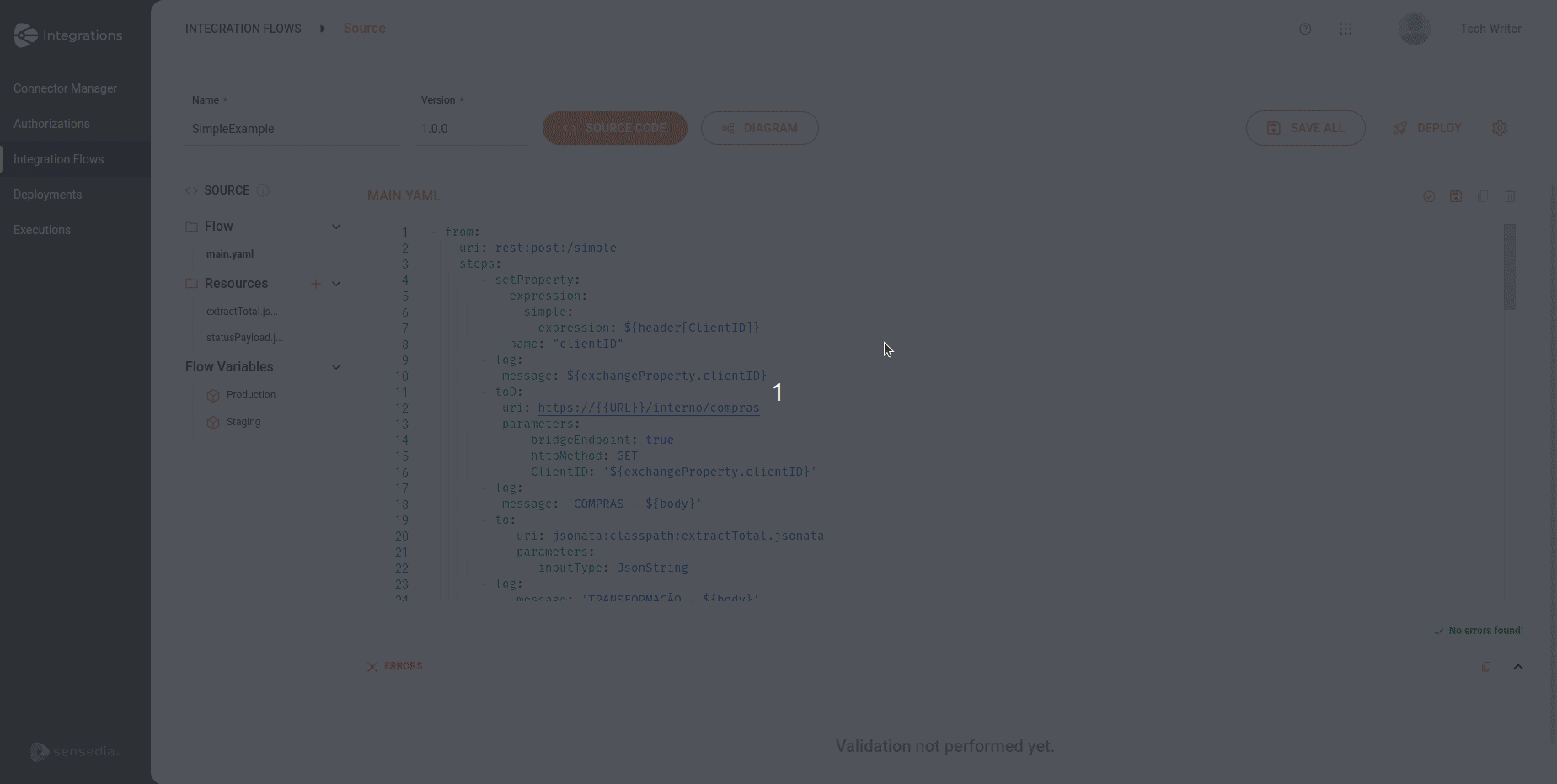
See below the description of the executed steps:
-
Order reception
The flow begins when an order is received with an identification code (ClientID). The
POST
request is forwarded to the/simple
endpoint. The response to the request is logged.- from: uri: rest:post:/simple - setProperty: expression: simple: expression: ${header[ClientID]} name: "clientID" - log: message: ${exchangeProperty.clientID}
-
Purchase information retrieval
With the ClientID, the system queries an internal service for more details. The
GET
request is made to the shopping endpoint, passing the ClientID as a parameter. The response to the request is logged.- toD: uri: https://{{URL}}/interno/compras parameters: bridgeEndpoint: true httpMethod: GET ClientID: '${exchangeProperty.clientID}' - log: message: 'COMPRAS - ${body}'
-
Data transformation
The received information is processed and formatted using a transformation tool, JSONata. The applied transformation is logged for verification.
- to: uri: jsonata:classpath:extractTotal.jsonata parameters: inputType: JsonString - log: message: 'TRANSFORMAÇÃO - ${body}'
-
The instructions for creating a new JSON object are in the
extractTotal.jsonata
file, available in the Resources folder. -
The
extractTotal.jsonata
file is referenced in the flow script along with the classpath (snippet above). -
Below is the content of the
extractTotal.jsonata
file:
{ "Conta": Cliente.Conta, "TipoPagamento": Cliente.TipoPagamento, "Valor": $sum(Cliente.Ordens.Produto.(Preco * Quantidade)) }
-
-
Payment processing
The processed data is then sent to an external service to make the payment. First, the transformed message body is converted to JSON format, and the
Content-Type
header (set to application/json) and theAuthorization
header (with an example token) are configured. APOST
request is then made to the payment endpoint. The received response is logged.- marshal: json: library: Jackson - setHeader: expression: constant: expression: application/json name: Content-Type - setHeader: expression: constant: expression: 'Bearer tokenDeAutorizacaoExemplo' name: Authorization - toD: uri: https://{{URL}}/externo/pagamento parameters: bridgeEndpoint: true httpMethod: POST - log: message: 'PAGAMENTO - ${body}'
-
Status update
After the payment is processed, the result is combined with the ClientID and transformed again to update the payment status in an internal service. The adjusted message body is converted to JSON format and sent in a
POST
request to the status endpoint.- setBody: expression: simple: expression: '{"ClientID": "${exchangeProperty.clientID}", "status":${body}}' - log: message: 'CONCATENAÇÃO - ${body}' - to: uri: jsonata:classpath:statusPayload.jsonata parameters: inputType: JsonString - log: message: 'STATUS PAYLOAD - ${body}' - marshal: json: library: Jackson - setHeader: expression: constant: expression: application/json name: Content-Type - toD: uri: https://{{URL}}/interno/status parameters: bridgeEndpoint: true httpMethod: POST
-
The instructions for creating a new JSON object are in the
statusPayload.jsonata
file, available in the Resources folder. -
The
statusPayload.jsonata
file is referenced in the flow script along with the classpath (snippet above). -
Below is the content of the
statusPayload.jsonata
file:
{ "ClientID": ClientID, "Status":status.status }
-
-
Final response
The system sends a final confirmation to indicate that the payment was successful.
The HTTP response code is set to 200
, and the response body confirms the success of the operation.
Additionally, the content of the final response is logged.
- setHeader:
expression:
constant:
expression: "200"
name: CamelHttpResponseCode
- setBody:
expression:
simple:
expression: '{"success": "true"}'
- log:
message: ${body}
Flow script
Below is the complete script for the described integration flow:
- from:
uri: rest:post:/simple
steps:
- setProperty:
expression:
simple:
expression: ${header[ClientID]}
name: "clientID"
- log:
message: ${exchangeProperty.clientID}
- toD:
uri: https://{{URL}}/interno/compras
parameters:
bridgeEndpoint: true
httpMethod: GET
ClientID: '${exchangeProperty.clientID}'
- log:
message: 'COMPRAS - ${body}'
- to:
uri: jsonata:classpath:extractTotal.jsonata
parameters:
inputType: JsonString
- log:
message: 'TRANSFORMAÇÃO - ${body}'
- marshal:
json:
library: Jackson
- setHeader:
expression:
constant:
expression: application/json
name: Content-Type
- setHeader:
expression:
constant:
expression: 'Bearer tokenDeAutorizacaoExemplo'
name: Authorization
- toD:
uri: https://{{URL}}/externo/pagamento
parameters:
bridgeEndpoint: true
httpMethod: POST
- log:
message: 'PAGAMENTO - ${body}'
- setBody:
expression:
simple:
expression: '{"ClientID": "${exchangeProperty.clientID}", "status":${body}}'
- log:
message: 'CONCATENAÇÃO - ${body}'
- to:
uri: jsonata:classpath:statusPayload.jsonata
parameters:
inputType: JsonString
- log:
message: 'STATUS PAYLOAD - ${body}'
- marshal:
json:
library: Jackson
- setHeader:
expression:
constant:
expression: application/json
name: Content-Type
- toD:
uri: https://{{URL}}/interno/status
parameters:
bridgeEndpoint: true
httpMethod: POST
- setHeader:
expression:
constant:
expression: "200"
name: CamelHttpResponseCode
- setBody:
expression:
simple:
expression: '{"success": "true"}'
- log:
message: ${body}
Share your suggestions with us!
Click here and then [+ Submit idea]